Python on Vercel
Introduction
This quickstart will guide you through setting up QStash to run a daily script to clean up your database. This is useful for testing and development environments where you want to reset the database every day.
Prerequisites
- Create an Upstash account and get your QStash token
Create Python app
First, we’ll create a new directory for our Python app. We’ll call it clean-db-cron
.
The database we’ll be using is Redis, so we’ll need to install the upstash_redis
package.
mkdir clean-db-cron
cd clean-db-cron
pip install upstash-redis
Cleanup logic
Let’s write the Python code to clean up the database. We’ll use the upstash_redis
package to connect to the database and delete all keys.
from upstash_redis import Redis
redis = Redis(url="https://YOUR_REDIS_URL", token="YOUR_TOKEN")
def delete_all_entries():
keys = redis.keys("*") # Match all keys
redis.delete(*keys)
delete_all_entries()
Try running the code to see if it works. Your database keys should be deleted!
Make the Python code into a public endpoint
In order to use QStash, we need to make the Python code into a public endpoint. There
are many ways to do this such as using Flask, FastAPI, or Django. In this example, we’ll
use the Python http.server
module to create a simple HTTP server.
from http.server import BaseHTTPRequestHandler
from upstash_redis import Redis
redis = Redis(url="https://YOUR_REDIS_URL", token="YOUR_TOKEN")
def delete_all_entries():
keys = redis.keys("*") # Match all keys
redis.delete(*keys)
class handler(BaseHTTPRequestHandler):
def do_POST(self):
delete_all_entries()
self.send_response(200)
self.end_headers()
For the purpose of this tutorial, I’ll deploy the application to Vercel using the Python Runtime, but feel free to use any other hosting provider.
Once deployed, you can find the public URL in the dashboard.
Have QStash invoke the endpoint
There are two ways we can go about configuring QStash. We can either use the QStash dashboard or the QStash API. In this example, it makes more sense to utilize the dashboard since we only need to set up a singular cronjob.
However, you can imagine a scenario where you have a large number of cronjobs and you’d want to automate the process. In that case, you’d want to use the QStash Python SDK.
To create the schedule, go to the QStash dashboard and enter the URL of the public endpoint you created. Then, set the schedule to run daily at a time of your choosing.
URL: https://your-vercel-app.vercel.app/api
Type: Scheduled
Every: every day at midnight (feel free to customize)
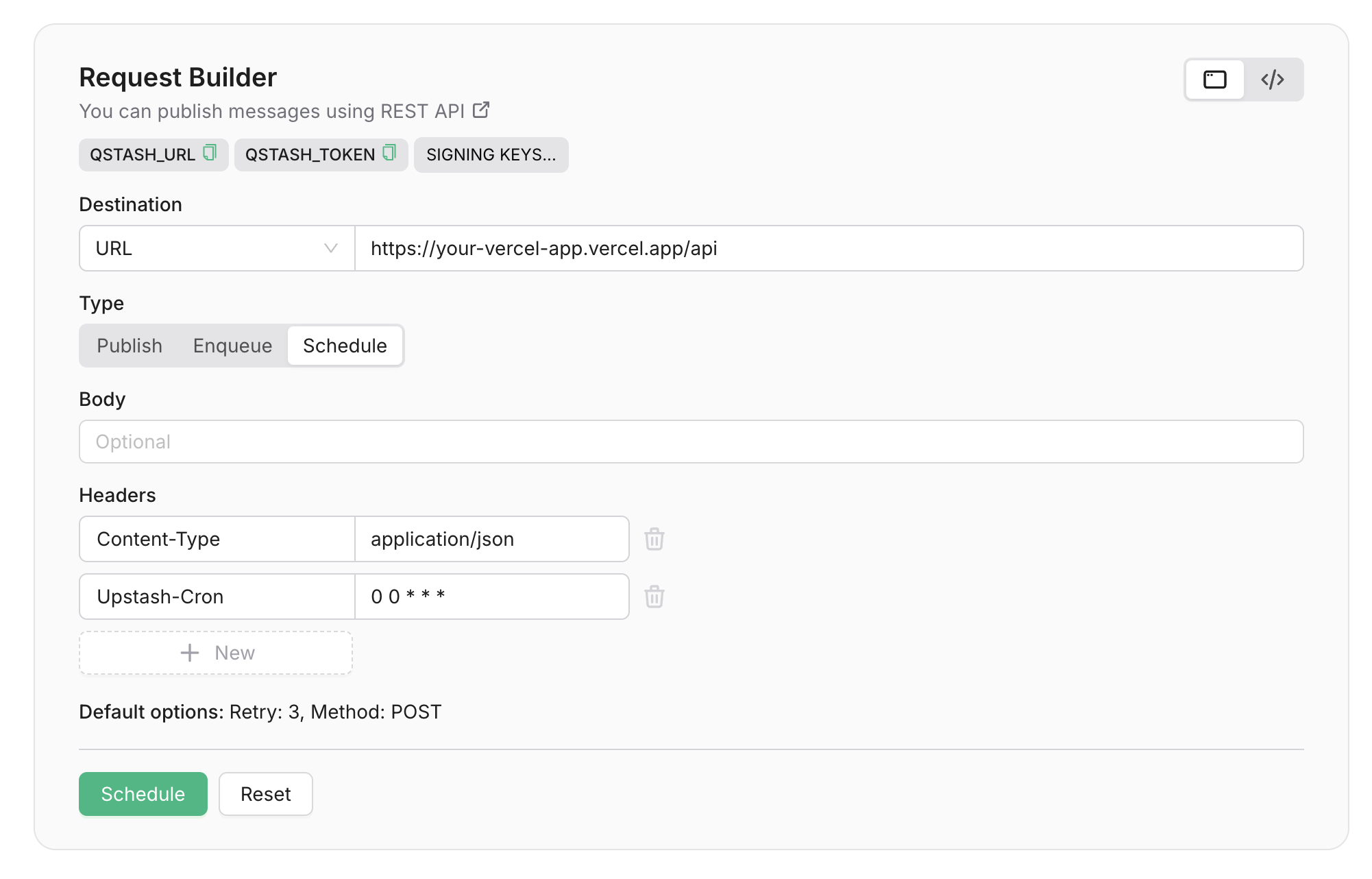
Once you start the schedule, QStash will invoke the endpoint at the specified time. You can scroll down and verify the job has been created!
Now, go ahead and try it out for yourself! Try using some of the other features of QStash, such as callbacks and topics.
Was this page helpful?