Background Jobs
When do you need background jobs
Background jobs are essential for executing tasks that are too time-consuming to run in the main execution thread without affecting the user experience.
These tasks might include data processing, sending batch emails, performing scheduled maintenance, or any other operations that are not immediately required to respond to user requests.
Utilizing background jobs allows your application to remain responsive and scalable, handling more requests simultaneously by offloading heavy lifting to background processes.
In Serverless frameworks, your hosting provider will likely have a limit for how long each task can last. Try searching for the maximum execution time for your hosting provider to find out more.
How to use QStash for background jobs
QStash provides a simple and efficient way to run background jobs, you can understand it as a 2 step process:
- Public API Create a public API endpoint within your application. The endpoint should contain the logic for the background job.
Since QStash requires a public endpoint to invoke the background job, it won’t be able to access any localhost APIs. To get around this, you can set up a local tunnel for local development.
- QStash Request Invoke QStash to start/schedule the execution of the API endpoint.
Here’s what this looks like in a simple Next.js application:
To better understand the application, let’s break it down:
- Client: The client application contains a button that, when clicked, sends a request to the server to start the background job.
- Next.js server: The first endpoint,
/api/start-email-job
, is invoked by the client to start the background job. - QStash: The QStash client is used to invoke the
/api/send-email
endpoint, which contains the logic for the background job.
Here is a visual representation of the process:
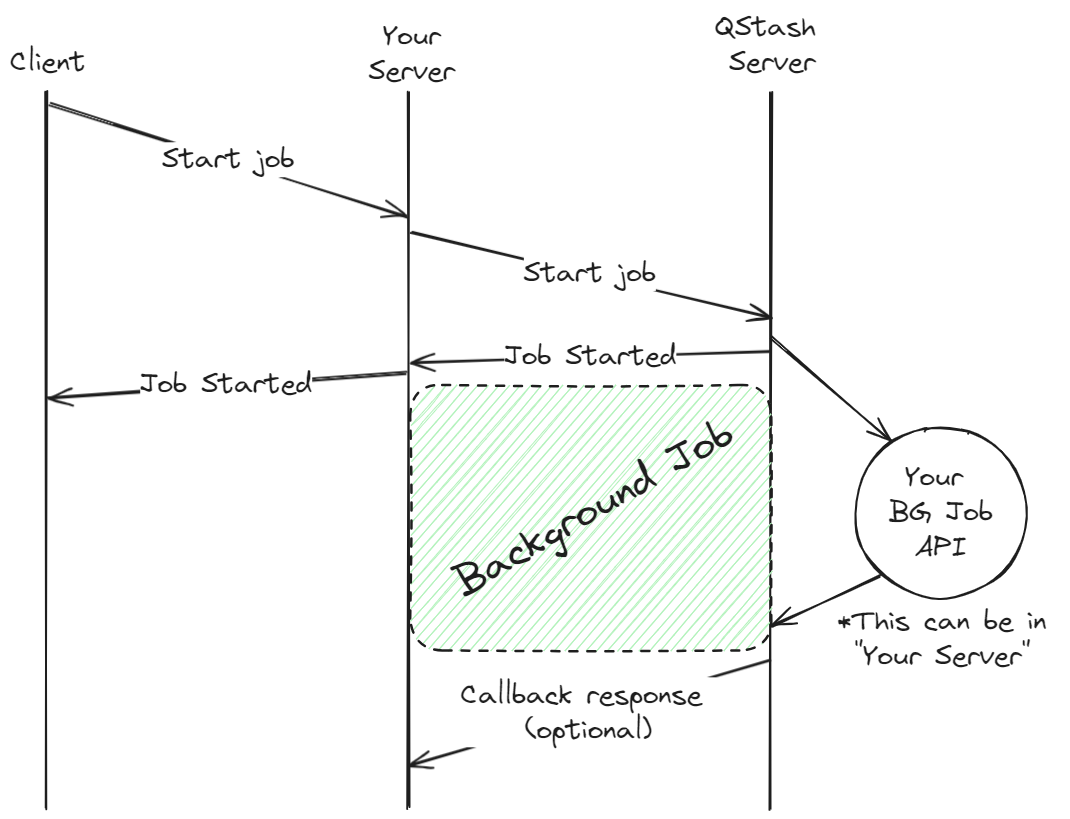
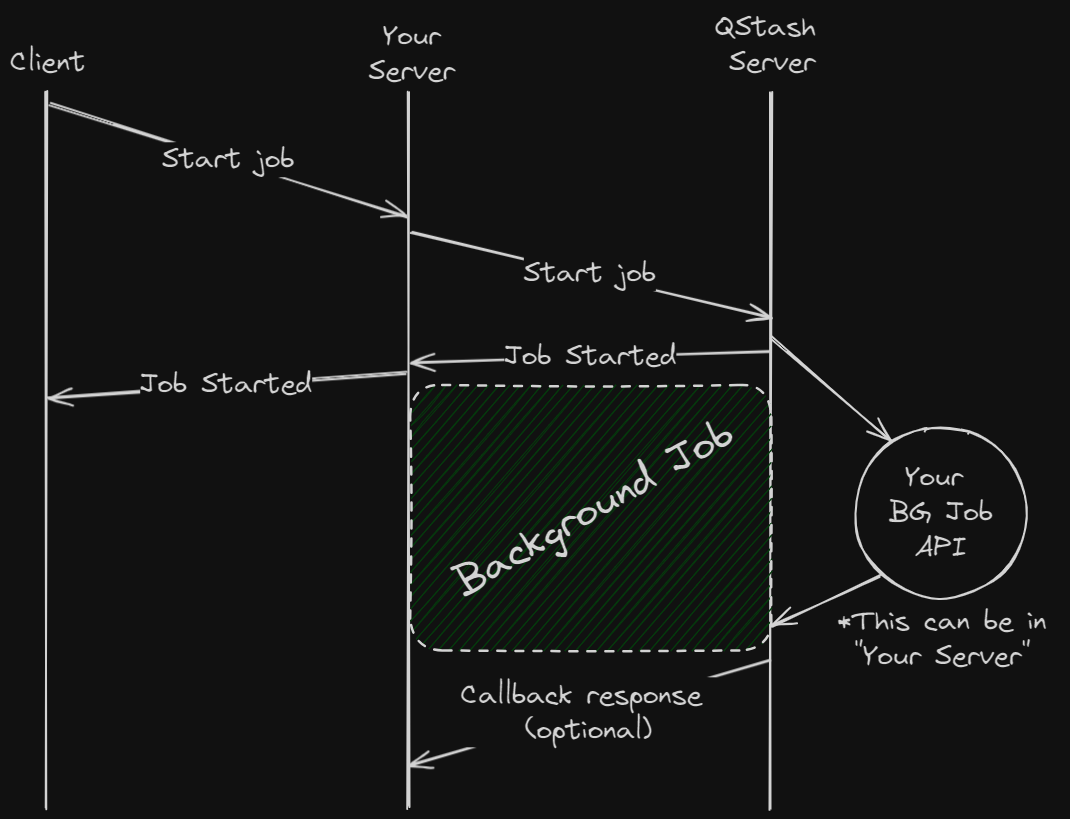
To view a more detailed Next.js quick start guide for setting up QStash, refer to the quick start guide.
It’s also possible to schedule a background job to run at a later time using schedules.
If you’d like to invoke another endpoint when the background job is complete, you can use callbacks.